Pointer Basics: Pointers and Memory, Pointer
Types
(ポインタの基礎:
ポインタとメモリ配置の関係、型としてのポインタ)
Computing Practice I
9th lecture, June 15, 2017
http://www.sw.it.aoyama.ac.jp/2017/CP1/lecture9.html
Martin J. Dürst

© 2005-17 Martin
J. Dürst 青山学院大学
Today's Schedule
- Minitest
- About last week's exam
- About last week's exercises
- Usages of pointers
- The structure of memory
- Operators for pointers
- Pointer types, declarations, and definitions
- Arrays and pointers
- Today's exercises
ミニテスト
- 授業開始までにログイン済み
- ナビゲーションは左に畳み、ブラウザは全画面に拡大
- 授業開始まで教科書、資料、筆箱、財布などを鞄に入れ、鞄を椅子の下に
- テスト終了後その場で待つ
Applications of C: Apache httpd
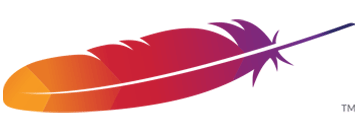
- Open source Web server
- First published in April 1995 as version 0.6.2, latest version 2.4.25
- From 1996 until today, uninterruptedly the most used Web serverる
- Current share: 35%~50% (deppending on statistic)
- Also used for rpcsr/moo/www.sw.it.aoyama.ac.jp
- About 240K lines of C code
Written Exam (two weeks ago)
- Maximum: 101 points
- Average: 59.5 points
- Best result: 98 points
- Worst result: 24 points
If you have less than 40points, please think very seriously how you can
improve.
Exams will be handed back after this lecture.
Results of Previous Exercises
|
08A1 |
08A2 |
08B1 |
08C1 |
08C2 |
100 points |
96 |
85 |
83 |
60 |
34 |
60 points |
2 |
13 |
15 |
35 |
59 |
errors |
- |
- |
- |
3 |
4 |
not submitted |
1 |
1 |
1 |
1 |
2 |
About Exercise 08C2
Important criterion for software: Extensibility
(for the Temperature class, e.g. adding a new temperature unit)
Choices for internal representation:
- Numeric value and unit
- One value for each of the three units
- Single value in single unit (example: Celsius)
Choices for processing:
- Conversion in setter
- Conversion in getter
- Conversion in both setter and getter
Because the internal details of the class are encapsulated, they don't
change the externally observable behavior.
Usage of Pointers
- Low-level address manipulation (memory-mapped devices,...)
- Array processing (incl. speedup)
- Dynamic memory management
- References (argument passing by reference,...など)
- Indirection
How Memory Works
- The (main) memory in the computer is a (huge) byte sequence
- 1 byte (=8 bits) is the smallest unit of memory
- Memory locations are identified by addresses
- Addresses are usually written as hexadecimal numbers
- People can handle names better than numbers.
Therefore, we usually use variable names,...
- Programs work internally with addresses, not names
- For data with lengths longer than a byte, the address of the lowest byte
is used
- Data with a size of 2/4/8 bytes is usually aligned to an address
divisible by the size of the data
- Variables that contain addresses are called pointers
Investigating Memory Layout
- To output pointers, use
%p
with printf
- We can output the addresses of various data items in a program
and visualize the layout of the data on a memory layout sheet
- Example: memory.c
Operators for Pointers (&
/*
)
- Address-of operator
&
(unary):
Take the address of a variable (e.g. to assign it to a pointer)
int count = 0, *pi;
pi = &count;
- Indirection operator (dereference operator)
*
(unary):
Find the data item pointed to by a pointer (i.e. dereference the
pointer)
printf("Count is %d.\n", *pi);
Pointer Types
- Pointers are separate from other types (integers, functions,
structures,...)
- Pointer types include the type of the referenced data item
- Examples: Pointer to
int
, pointer to
double
,
pointer to char
, pointer to a structure
Person
- This decides on the type of the result of a
*
operation
Example: If pi
is a pointer to int
, then
*pi
is of type int
- This avoids some kind of mistakes (pointing to the wrong data)
Declarations and Definitions of Pointers
- Add a
*
before the variable name and make the type being
pointed to explicit
- The priority of 'operators' in declarations/definitions is the same as
the priority in expressions (see p. 388)
int *pi;
can be read as "pi
is a pointer to an
integer", but also as "*pi
is an integer", or
"pi
, when dereferenced, is an integer
Examples of Pointer Definitions
int *pi; // pointer to int
char *pc; // pointer to char(acter)
// also pointer to (the start of a) character string
int **ppi; // pointer to pointer to int
char a[5]; // array of 5 characters
char *pb[5]; // char *(pb[5])
// array of length 5 of pointers to char(acters)
char (*pc)[5]; // pointer to a (character) string of length 5
char c, a[5], *pc, *pa[5], (*pb)[5]; // only char applies to everything
(see also http://ieng9.ucsd.edu/~cs30x/rt_lt.rule.html;
challenge: implement as program)
Operators for Pointers (+
/-
)
- Integers can be added or subtracted to/from pointers
- Addition, subtraction (
p+5
, p-3
,...)
- Combination with assignment (
p += 7
,...)
- Increment, decrement (
p++
, p--
)
- For an integer of 1, the pointer moves by one time the length of the type
being pointed to
(the type being pointed to is the unit of length used (cf. mm, cm, m,
km))
- Example:
printf("size: %d, address: %p (%d)", sizeof(*pi), pi, pi);
→ size: 8, address: 0x220c8 (139464)
pi += 10;
printf("address: %p (%d)", pi, pi);
→ address: 0x22118 (139544)
- It is possible to subtract two pointers of the same type from each other.
The result is an integer.
- Attention:
*p++
and (*p)++
are different (see
p. 388)
Arrays and Pointers
- In an array, data items of the same type follow each other
- C only remembers the location of the first array element
- Arrays and pointers are very similar (almost identical)
- Exception: In the scope (function) where an array is declared
- Assignment to the array itself is prohibited
sizeof
returns the size of the whole array
Example of memory layout for arrays and
pointers
Explanation about Experiment
- Idea: Provide hints for improvement based on past years' attempts
- This week: Preliminary tests
- From next week: Actual experiment (more detailled explanations, consent
form)
- Line with
------
may be missing
- Lines with light blue
background may need changes
- Hints may be correct, or may be wrong, or may be missing
演習問題について
- 09A1: 各データ型のポインタ・アドレス
(型の順番、変数名の変更に注意)
- 09A2: ポインタの動きの予想・観察
(
++
/--
)
- 09A3: ポインタと配列の関係
- 09B1: 大文字を数える関数 (Improvement
Hints あり)
- 09C1: 月の番号を英語名に変更 (英語以外も可能; 09C2
も同様)
- 09C2: メモリ配置表の記入
注: 重要: 全問必須
(全員提出)、全問日曜締切
09A2, 09A3, 09C2:
プログラムをシステムに提出、かつ用紙を先生に提出
(09C2 の用紙の最後の締め切りは次回の演習開始)
次回の準備
- 宿題として残った問題を完成、提出
- 今日の復習
- 「ポインタが理解できない理由」の第六章 「malloc
とポインタ」(浅井 淳、技術評論社、2002)
をよく読む。
- 参考書の第 11 章 (ポインタ、pp. 282-311)
をもう一回よく読む。分かるところをリストアップする。
Glossary
- pointer
- ポインタ
- address manipulation
- アドレス操作
- dynamic memory
- 動的メモリ
- reference
- 参照
- argument passing by reference
- 関数への参照渡し
- indirection
- 間接
- extensibility
- 拡張性
- unit
- 単位
- byte sequence
- バイト列
- byte
- バイト
- bit
- ビット
- address
- 番地、アドレス
- address-of operator
- アドレス演算子
- unary
- 単項
- indirection operator (dereference operator)
- 間接演算子
- scope
- スコープ、有効範囲